mirror of
https://github.com/python-kasa/python-kasa.git
synced 2024-12-23 11:43:34 +00:00
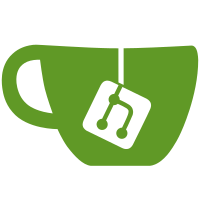
Re-query failed modules after some delay instead of immediately disabling them. Changes to features so they can still be created when modules are erroring.
61 lines
1.7 KiB
Python
61 lines
1.7 KiB
Python
"""Implementation of humidity module."""
|
|
|
|
from __future__ import annotations
|
|
|
|
from typing import TYPE_CHECKING
|
|
|
|
from ...feature import Feature
|
|
from ..smartmodule import SmartModule
|
|
|
|
if TYPE_CHECKING:
|
|
from ..smartdevice import SmartDevice
|
|
|
|
|
|
class HumiditySensor(SmartModule):
|
|
"""Implementation of humidity module."""
|
|
|
|
REQUIRED_COMPONENT = "humidity"
|
|
QUERY_GETTER_NAME = "get_comfort_humidity_config"
|
|
|
|
def __init__(self, device: SmartDevice, module: str):
|
|
super().__init__(device, module)
|
|
self._add_feature(
|
|
Feature(
|
|
device,
|
|
id="humidity",
|
|
name="Humidity",
|
|
container=self,
|
|
attribute_getter="humidity",
|
|
icon="mdi:water-percent",
|
|
unit_getter=lambda: "%",
|
|
category=Feature.Category.Primary,
|
|
type=Feature.Type.Sensor,
|
|
)
|
|
)
|
|
self._add_feature(
|
|
Feature(
|
|
device,
|
|
id="humidity_warning",
|
|
name="Humidity warning",
|
|
container=self,
|
|
attribute_getter="humidity_warning",
|
|
type=Feature.Type.BinarySensor,
|
|
icon="mdi:alert",
|
|
category=Feature.Category.Debug,
|
|
)
|
|
)
|
|
|
|
def query(self) -> dict:
|
|
"""Query to execute during the update cycle."""
|
|
return {}
|
|
|
|
@property
|
|
def humidity(self):
|
|
"""Return current humidity in percentage."""
|
|
return self._device.sys_info["current_humidity"]
|
|
|
|
@property
|
|
def humidity_warning(self) -> bool:
|
|
"""Return true if humidity is outside of the wanted range."""
|
|
return self._device.sys_info["current_humidity_exception"] != 0
|