mirror of
https://github.com/python-kasa/python-kasa.git
synced 2024-12-23 19:53:34 +00:00
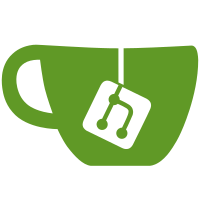
Address the inconsistent naming of smart modules by removing all "Module" suffixes and aligning filenames with class names. Removes the casting of modules to the correct module type now that is is redundant. Update the adding of iot modules to use the ModuleName class rather than a free string.
43 lines
1.1 KiB
Python
43 lines
1.1 KiB
Python
"""Implementation of contact sensor module."""
|
|
|
|
from __future__ import annotations
|
|
|
|
from typing import TYPE_CHECKING
|
|
|
|
from ...feature import Feature
|
|
from ..smartmodule import SmartModule
|
|
|
|
if TYPE_CHECKING:
|
|
from ..smartdevice import SmartDevice
|
|
|
|
|
|
class ContactSensor(SmartModule):
|
|
"""Implementation of contact sensor module."""
|
|
|
|
REQUIRED_COMPONENT = None # we depend on availability of key
|
|
REQUIRED_KEY_ON_PARENT = "open"
|
|
|
|
def __init__(self, device: SmartDevice, module: str):
|
|
super().__init__(device, module)
|
|
self._add_feature(
|
|
Feature(
|
|
device,
|
|
id="is_open",
|
|
name="Open",
|
|
container=self,
|
|
attribute_getter="is_open",
|
|
icon="mdi:door",
|
|
category=Feature.Category.Primary,
|
|
type=Feature.Type.BinarySensor,
|
|
)
|
|
)
|
|
|
|
def query(self) -> dict:
|
|
"""Query to execute during the update cycle."""
|
|
return {}
|
|
|
|
@property
|
|
def is_open(self):
|
|
"""Return True if the contact sensor is open."""
|
|
return self._device.sys_info["open"]
|