mirror of
https://github.com/python-kasa/python-kasa.git
synced 2024-12-23 11:43:34 +00:00
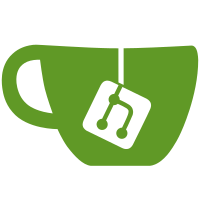
Addresses stability issues on older hw device versions - Handles module timeout errors better by querying modules individually on errors and disabling problematic modules like Firmware that go out to the internet to get updates. - Addresses an issue with the Led module on P100 hardware version 1.0 which appears to have a memory leak and will cause the device to crash after approximately 500 calls. - Delays updates of modules that do not have regular changes like LightPreset and LightEffect and enables them to be updated on the next update cycle only if required values have changed.
50 lines
1.4 KiB
Python
50 lines
1.4 KiB
Python
"""Implementation of cloud module."""
|
|
|
|
from __future__ import annotations
|
|
|
|
from typing import TYPE_CHECKING
|
|
|
|
from ...feature import Feature
|
|
from ..smartmodule import SmartModule
|
|
|
|
if TYPE_CHECKING:
|
|
from ..smartdevice import SmartDevice
|
|
|
|
|
|
class Cloud(SmartModule):
|
|
"""Implementation of cloud module."""
|
|
|
|
QUERY_GETTER_NAME = "get_connect_cloud_state"
|
|
REQUIRED_COMPONENT = "cloud_connect"
|
|
MINIMUM_UPDATE_INTERVAL_SECS = 60
|
|
|
|
def _post_update_hook(self):
|
|
"""Perform actions after a device update.
|
|
|
|
Overrides the default behaviour to disable a module if the query returns
|
|
an error because the logic here is to treat that as not connected.
|
|
"""
|
|
|
|
def __init__(self, device: SmartDevice, module: str):
|
|
super().__init__(device, module)
|
|
|
|
self._add_feature(
|
|
Feature(
|
|
device,
|
|
id="cloud_connection",
|
|
name="Cloud connection",
|
|
container=self,
|
|
attribute_getter="is_connected",
|
|
icon="mdi:cloud",
|
|
type=Feature.Type.BinarySensor,
|
|
category=Feature.Category.Info,
|
|
)
|
|
)
|
|
|
|
@property
|
|
def is_connected(self):
|
|
"""Return True if device is connected to the cloud."""
|
|
if self._has_data_error():
|
|
return False
|
|
return self.data["status"] == 0
|