mirror of
https://github.com/python-kasa/python-kasa.git
synced 2025-07-02 01:29:55 +00:00
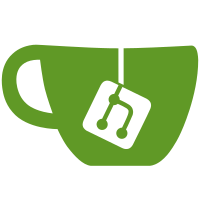
Python 3.11 ships with latest Debian Bookworm. pypy is not that widely used with this library based on statistics. It could be added back when pypy supports python 3.11.
49 lines
1.6 KiB
Python
49 lines
1.6 KiB
Python
"""Original implementation of the TP-Link Smart Home protocol."""
|
|
|
|
import struct
|
|
from collections.abc import Generator
|
|
|
|
|
|
class OriginalTPLinkSmartHomeProtocol:
|
|
"""Original implementation of the TP-Link Smart Home protocol."""
|
|
|
|
INITIALIZATION_VECTOR = 171
|
|
|
|
@staticmethod
|
|
def _xor_payload(unencrypted: bytes) -> Generator[int, None, None]:
|
|
key = OriginalTPLinkSmartHomeProtocol.INITIALIZATION_VECTOR
|
|
for unencryptedbyte in unencrypted:
|
|
key = key ^ unencryptedbyte
|
|
yield key
|
|
|
|
@staticmethod
|
|
def encrypt(request: str) -> bytes:
|
|
"""Encrypt a request for a TP-Link Smart Home Device.
|
|
|
|
:param request: plaintext request data
|
|
:return: ciphertext to be send over wire, in bytes
|
|
"""
|
|
plainbytes = request.encode()
|
|
return struct.pack(">I", len(plainbytes)) + bytes(
|
|
OriginalTPLinkSmartHomeProtocol._xor_payload(plainbytes)
|
|
)
|
|
|
|
@staticmethod
|
|
def _xor_encrypted_payload(ciphertext: bytes) -> Generator[int, None, None]:
|
|
key = OriginalTPLinkSmartHomeProtocol.INITIALIZATION_VECTOR
|
|
for cipherbyte in ciphertext:
|
|
plainbyte = key ^ cipherbyte
|
|
key = cipherbyte
|
|
yield plainbyte
|
|
|
|
@staticmethod
|
|
def decrypt(ciphertext: bytes) -> str:
|
|
"""Decrypt a response of a TP-Link Smart Home Device.
|
|
|
|
:param ciphertext: encrypted response data
|
|
:return: plaintext response
|
|
"""
|
|
return bytes(
|
|
OriginalTPLinkSmartHomeProtocol._xor_encrypted_payload(ciphertext)
|
|
).decode()
|