mirror of
https://github.com/gnif/LookingGlass.git
synced 2025-07-07 03:59:52 +00:00
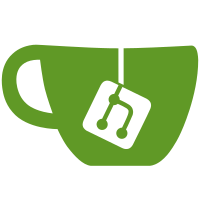
We are actually getting mouse events directly from Wayland instead of going through SDL, so we call app_updateCursorPos in pointer motion handlers and swallow the SDL event. Also removed parameters for app_handleMouseBasic as it relies exclusively on absolute positions provided by app_updateCursorPos. Wayland does not give you relative movements at all unless grabbed and passing absolute movements is semantically incorrect. Note that when the cursor is grabbed, movements are handled entirely through relativePointerMotionHandler in wayland.c and does not go through app_handleMouseBasic at all.
45 lines
1.6 KiB
C
45 lines
1.6 KiB
C
/*
|
|
Looking Glass - KVM FrameRelay (KVMFR) Client
|
|
Copyright (C) 2017-2020 Geoffrey McRae <geoff@hostfission.com>
|
|
https://looking-glass.hostfission.com
|
|
|
|
This program is free software; you can redistribute it and/or modify it under
|
|
the terms of the GNU General Public License as published by the Free Software
|
|
Foundation; either version 2 of the License, or (at your option) any later
|
|
version.
|
|
|
|
This program is distributed in the hope that it will be useful, but WITHOUT ANY
|
|
WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A
|
|
PARTICULAR PURPOSE. See the GNU General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License along with
|
|
this program; if not, write to the Free Software Foundation, Inc., 59 Temple
|
|
Place, Suite 330, Boston, MA 02111-1307 USA
|
|
*/
|
|
|
|
#include <stdbool.h>
|
|
|
|
#include "interface/displayserver.h"
|
|
|
|
|
|
SDL_Window * app_getWindow(void);
|
|
|
|
bool app_getProp(LG_DSProperty prop, void * ret);
|
|
bool app_inputEnabled(void);
|
|
bool app_cursorIsGrabbed(void);
|
|
bool app_cursorWantsRaw(void);
|
|
bool app_cursorInWindow(void);
|
|
void app_updateCursorPos(double x, double y);
|
|
void app_updateWindowPos(int x, int y);
|
|
void app_handleResizeEvent(int w, int h);
|
|
void app_handleMouseGrabbed(double ex, double ey);
|
|
void app_handleMouseNormal(double ex, double ey);
|
|
void app_handleMouseBasic(void);
|
|
void app_handleWindowEnter(void);
|
|
void app_handleWindowLeave(void);
|
|
|
|
void app_clipboardRelease(void);
|
|
void app_clipboardNotify(const LG_ClipboardData type, size_t size);
|
|
void app_clipboardData(const LG_ClipboardData type, uint8_t * data, size_t size);
|
|
void app_clipboardRequest(const LG_ClipboardReplyFn replyFn, void * opaque);
|