mirror of
https://github.com/gnif/LookingGlass.git
synced 2024-09-20 09:21:33 +00:00
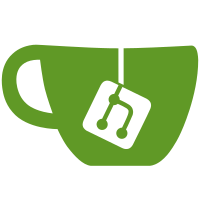
According to Erik @ NVidia the open source NVidia driver will not create a EGLImage from a DMABUF if the target is not GL_TEXTURE_EXTERNAL_OES. This change set converts the dmabuf texture from GL_TEXTURE_2D to GL_TEXTURE_EXTERNAL_OES and at runtime performs a global search & replace on fragment shaders as needed to remain compatible, replacing `sampler2D` with `samplerExternalOES`. Ref: https://github.com/NVIDIA/open-gpu-kernel-modules/discussions/243#discussioncomment-3283415
63 lines
1.2 KiB
GLSL
63 lines
1.2 KiB
GLSL
#version 300 es
|
|
#extension GL_OES_EGL_image_external_essl3 : enable
|
|
|
|
precision highp float;
|
|
|
|
#define PI 3.141592653589793
|
|
|
|
in vec2 fragCoord;
|
|
out vec4 fragColor;
|
|
|
|
uniform sampler2D sampler1;
|
|
float sinc(float x)
|
|
{
|
|
return x == 0.0 ? 1.0 : sin(x * PI) / (x * PI);
|
|
}
|
|
|
|
float lanczos(float x)
|
|
{
|
|
return sinc(x) * sinc(x * 0.5);
|
|
}
|
|
|
|
float lanczos(vec2 v)
|
|
{
|
|
return lanczos(v.x) * lanczos(v.y);
|
|
}
|
|
|
|
void main()
|
|
{
|
|
vec2 size = vec2(textureSize(sampler1, 0));
|
|
vec2 pos = fragCoord * size;
|
|
vec2 invSize = 1.0 / size;
|
|
vec2 uvc = floor(pos) + vec2(0.5, 0.5);
|
|
|
|
vec2 uvs[9] = vec2[](
|
|
uvc + vec2(-1.0, -1.0),
|
|
uvc + vec2(-1.0, 0.0),
|
|
uvc + vec2(-1.0, 1.0),
|
|
uvc + vec2( 0.0, -1.0),
|
|
uvc + vec2( 0.0, 0.0),
|
|
uvc + vec2( 0.0, 1.0),
|
|
uvc + vec2( 1.0, -1.0),
|
|
uvc + vec2( 1.0, 0.0),
|
|
uvc + vec2( 1.0, 1.0)
|
|
);
|
|
|
|
float factors[9];
|
|
float sum = 0.0;
|
|
for (int i = 0; i < 9; ++i)
|
|
{
|
|
factors[i] = lanczos(uvs[i] - fragCoord * size);
|
|
sum += factors[i];
|
|
}
|
|
|
|
for (int i = 0; i < 9; ++i)
|
|
factors[i] /= sum;
|
|
|
|
vec3 color = vec3(0.0);
|
|
for (int i = 0; i < 9; ++i)
|
|
color += texture(sampler1, uvs[i] * invSize).rgb * factors[i];
|
|
|
|
fragColor = vec4(color, 1.0);
|
|
}
|